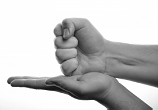
Angular
0
Answer link
After updating angular to the latest version, you might get this error:
Argument of type 'MonoTypeOperatorFunction<RouterEvent>' is not assignable to parameter of type 'OperatorFunction<Event_2, RouterEvent>'.
Types of parameters 'source' and 'source' are incompatible.
Type 'Observable<Event_2>' is not assignable to type 'Observable<RouterEvent>'.
Type 'Event_2' is not assignable to type 'RouterEvent'.
Type 'RouteConfigLoadStart' is missing the following properties from type 'RouterEvent': id, url
This error occurs at line where to subscribe to router events, that looks something like this:
this.routeSub = this.router.events
.pipe(
filter(
(event: RouterEvent) => event instanceof NavigationStart
),
tap(() => this.trigger.closePanel())
)
.subscribe();
Solution 1:
In recent version of angular, router.events emit a generic Event and not RouterEvent. Hence to correctly fix the issue import the Event from the angular/router module and replace RouterEvent with Event.
Here is how you do it:
Here is how you do it:
import { NavigationStart, Router, Event} from "@angular/router";
this.routeSub = this.router.events
.pipe(
filter(
(event: Event) => event instanceof NavigationStart
),
tap(() => this.trigger.closePanel())
)
.subscribe();
Solution 2:
If you really don't care about explicit type(e.g. in the filter function above), just remove the RouterEvent type, and compiler will implicitly assign the Event type to it.