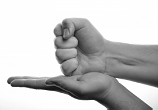
Web Development
A leading front-end development framework is React.
Here's why React is considered a leading framework:
-
Component-Based Architecture: React allows developers to build reusable UI components, making it easier to manage and scale applications.
-
Virtual DOM: React uses a virtual DOM to optimize updates to the actual DOM, improving performance.
-
Large Community and Ecosystem: React has a massive community, which provides extensive support, libraries, and tools.
-
Declarative Syntax: React uses a declarative syntax, making code easier to understand and maintain.
-
Cross-Platform Development: With frameworks like React Native, developers can use their React knowledge to build mobile applications.
You can learn more about React at its official website.
Here is how you do it:
Yes, it is possible to change the HTML tag of the string within the second column (td
) to another HTML tag like span
. This can be accomplished using various methods, depending on the context in which you're working, such as:
1. JavaScript/DOM Manipulation:
-
Explanation: You can use JavaScript to select the
td
element and then replace its content with aspan
element containing the same text. -
Example:
<table id="myTable"> <tr> <td>Column 1</td> <td>Old Content</td> <td>Column 3</td> </tr> </table> <script> const table = document.getElementById('myTable'); const secondCell = table.rows[0].cells[1]; // Get the second cell in the first row const span = document.createElement('span'); span.textContent = secondCell.textContent; // Copy the text content secondCell.innerHTML = ''; // Clear the cell content secondCell.appendChild(span); // Append the span to the cell </script>
2. Server-Side Rendering (e.g., PHP, Python, Node.js):
-
Explanation: If you're generating the HTML dynamically on the server, you can modify the code that generates the table to output a
span
instead of atd
for the second column. -
Example (PHP):
<table> <tr> <td>Column 1</td> <td><span><?php echo $data; ?></span></td> <td>Column 3</td> </tr> </table>
3. Using a Templating Engine (e.g., React, Angular, Vue.js):
-
Explanation: If you're using a templating engine, you can conditionally render a
span
instead of atd
based on your application's logic. -
Example (React):
function MyComponent(props) { return ( <table> <tr> <td>Column 1</td> <td><span>{props.data}</span></td> <td>Column 3</td> </tr> </table> ); }
The best approach depends on how your HTML is being generated and the specific requirements of your application. If the HTML is static, JavaScript is the easiest way to modify it. If the HTML is dynamically generated on the server, modify the server-side code. If you are using a front-end framework like React, Angular, or Vue.js, modify the component that renders the table.